In this tutorial, we will study how to update the records of the table using the Nodejs application.
Update the table from NodeJS program
To update the records in the table, the UPDATE statement will be used. We will update the table using the update.js file. The javascript code for the following is:
var mysql = require('mysql');
var con = mysql.createConnection({
host: "localhost",
user: "root",
password: "******",
database: "app"
});
con.connect(function(err) {
if (err) throw err;
var sql = "UPDATE info SET Name = 'Nupur' WHERE Name = 'Peter'";
con.query(sql, function (err, result) {
if (err) throw err;
console.log(result.affectedRows + " record(s) updated");
});
});
Code language: JavaScript (javascript)
Here we have used the UPDATE statement and in the callback function result.affectedRows property is used which will return the rows which are updated or modified.
Let us check the result set before modifying the record-
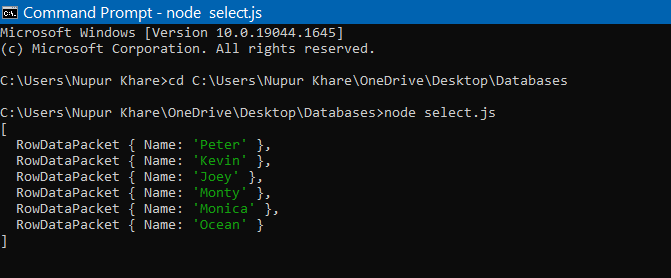
Now run the below command to get the result-
node update.js
Code language: SQL (Structured Query Language) (sql)
Output-
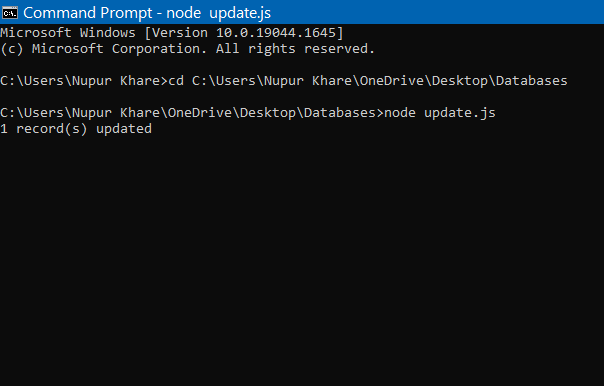
Now let us verify whether the changes have been made in the table or not. For this run the below query-
Select * from info;
Code language: SQL (Structured Query Language) (sql)
Output-
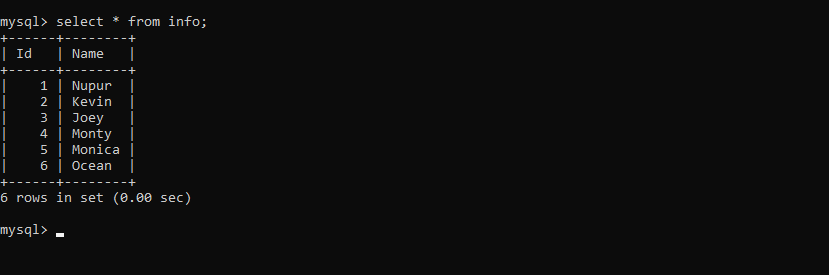
The first record that was earlier ‘Peter’ is now updated and changed to ‘Nupur’. When we execute the UPDATE statement, data is passed to the update statement in the form of an array. So when the changes will be passed the id will be set to 1 and as soon as the changes are made the id will be set to 0 which is false in Nodejs.
Conclusion
In this tutorial, we studied how to update the records in the table from the Nodejs application.