While dealing with a vast amount of data, there’s often a need to fetch the top value of the database, and going through the entire dataset for this procedure can be time-consuming. That’s where MySQL LIMIT and BETWEEN come into the picture. It allows users to extract the cream of the crop without sifting through the entire dataset. Whether you are a MySQL developer or a data analyst, this tool is a complete game changer. Although they both serve distinct purposes, understanding their difference is also important. Let’s get started!
An Overview of LIMIT Clause
Imagine a scenario where you are dealing with a big list of data, like sales records. The LIMIT clause helps you to pick out just the first few items from that list. It’s like saying “Get me the record of the top 5 sales figures”.
This clause is used to fetch a specific number of records from the top of the result set.
Syntax
SELECT column1, column2, ...
FROM table
ORDER BY column1, column2, ...
LIMIT number_of_rows;
Code language: SQL (Structured Query Language) (sql)
Now look at the below table, we are going to use this for the rest of our examples.
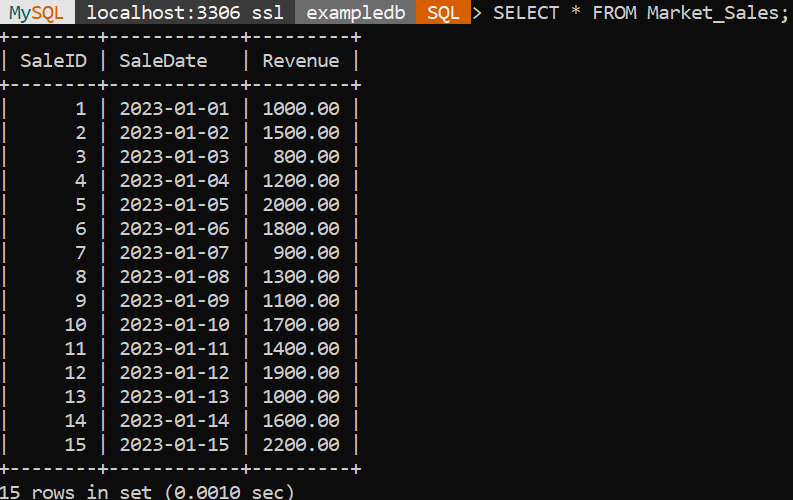
Example 1: Fetching Top Records
Let’s say we want to fetch the top five records from the Market_Sales table.
SELECT *
FROM Market_Sales
ORDER BY SaleDate
LIMIT 5;
Code language: SQL (Structured Query Language) (sql)
Output:
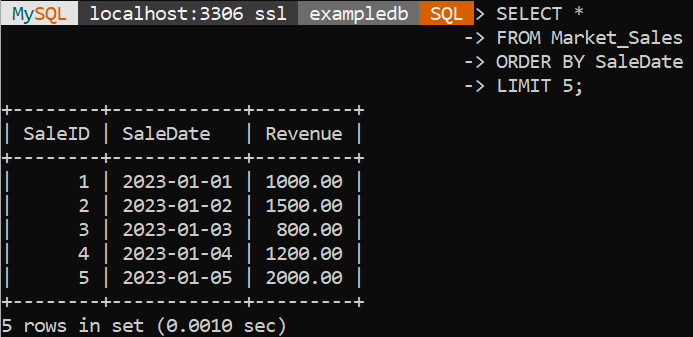
Example 2: Pagination
Now, if you want to implement pagination to fetch the next five orders, from the Market_Sales table.
SELECT *
FROM Market_Sales
ORDER BY SaleDate
LIMIT 5,5;
Code language: SQL (Structured Query Language) (sql)
Output:
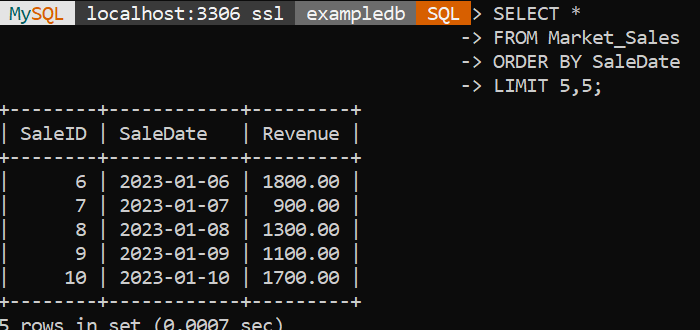
An Overview of BETWEEN Clause
Let’s say you have that same big list of sales records. The BETWEEN clause allows you to pick out numbers within a certain range. It’s like saying, “Show me the sales record where the revenue is between 1000 and 1200.”
It allows you to filter results based on a specific range of values.
Syntax
SELECT column1, column2, ...
FROM table
WHERE column_name BETWEEN value1 AND value2;
Code language: SQL (Structured Query Language) (sql)
Example 1: Filtering by Date Range
If we want to fetch the record of sales record from the date “01/01/2023” to “04/01/23” from the Market_Sales table.
SELECT *
FROM Market_Sales
WHERE SaleDate BETWEEN '2023-01-01' AND '2023-01-04';
Code language: SQL (Structured Query Language) (sql)
Output:
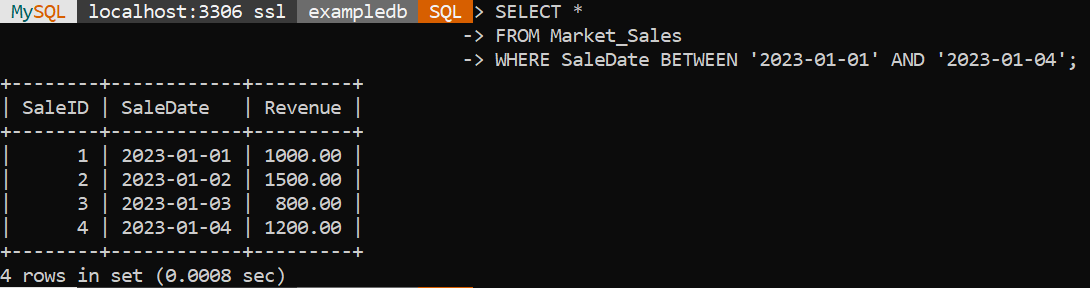
Example 2: Filtering by Numeric Range
If we want to fetch the record of sales record whose revenue is between 1000 and 2000 from the Market_Sales table.
SELECT *
FROM Market_Sales
WHERE Revenue BETWEEN 1000 AND 12000;
Code language: SQL (Structured Query Language) (sql)
Output:
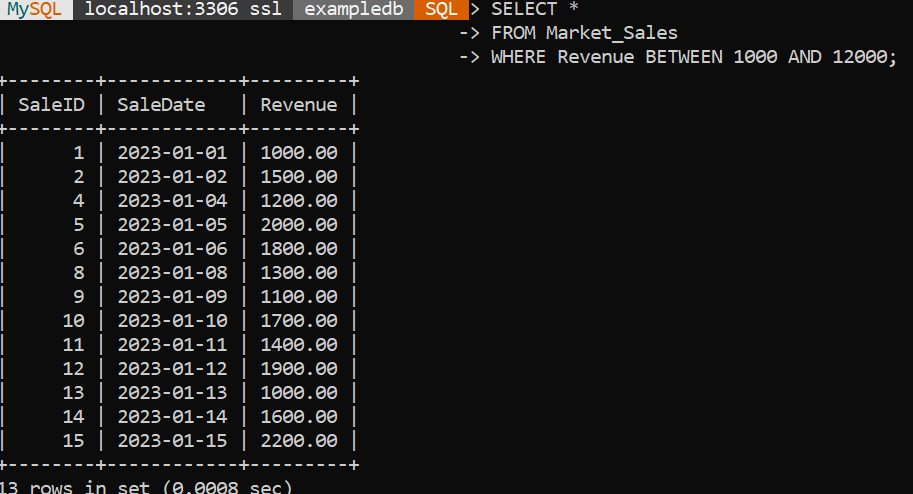
Filtering Data Using LIMIT and BETWEEN
Let’s combine the usage of both the LIMIT and BETWEEN clauses. If we want to fetch the top 5 highest revenue sales made between January 1, 2023, and January 12, 2023, from the table Market_Sales:
SELECT *
FROM Market_Sales
WHERE SaleDate BETWEEN '2023-01-01' AND '2023-04-12'
ORDER BY Revenue DESC
LIMIT 5;
Code language: SQL (Structured Query Language) (sql)
Output:
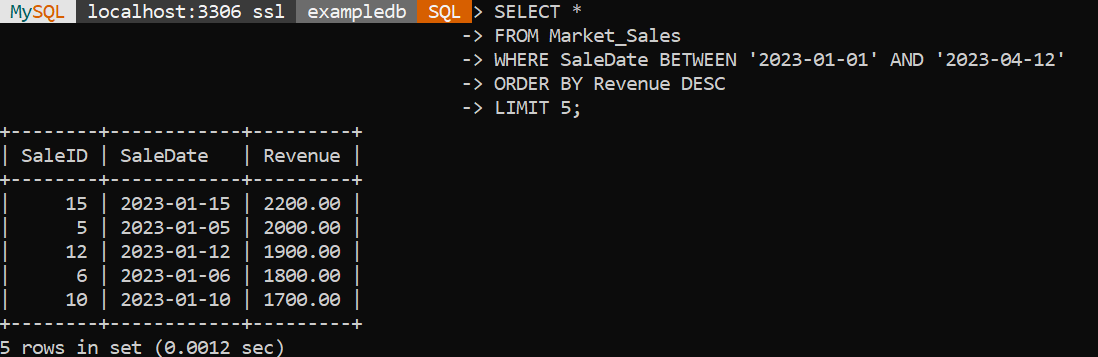
Also Read: Filtering Data with WHERE & HAVING Clause
Choosing Between SQL LIMIT and BETWEEN
When you are deciding between the MySQL LIMIT and BETWEEN clauses, consider these factors:
Factors | LIMIT | BETWEEN |
Purpose | Restrict the number of rows which are returned by a query, mainly for pagination or to fetch top/bottom records. | Inclusive (includes value between certain ranges). |
Usage | It may require appropriate indexing for performance. | Used to filter rows based on a specified range. |
Data Types | Applies to result set rows. | Applies to roes containing comparable values. |
Inclusivity | Not applicable (only specific row count). | It may require appropriate indexing for performance. |
Performance | Improves query performance by limiting result size. | May require appropriate indexing for performance. |
Conclusion
Data filtering from a vast amount of data can be a complex task and to make things easier we can use the tools provided by MySQL which are LIMIT and BETWEEN clauses. It will increase the efficiency as well as performance of the model. In this tutorial, we have learned these clauses to understand data manipulation better. And if you are dealing with a chunk of data, you must use this tool to speed up your work. We hope you enjoyed it.
Reference
https://stackoverflow.com/questions/4708708/how-does-mysql-process-order-by-and-limit-in-a-query