In this tutorial, we will learn how to query data in a table from the Nodejs application by connecting to the MySQL database server.
Selecting data from a MySQL table using NodeJS
To select all the data from the table, the SELECT statement will be used. Here we will use the database named app which has the table info and query the data from it. The javascript code for the following is-
var mysql = require('mysql');
var con = mysql.createConnection({
host: "localhost",
user: "root",
password: "*****",
database: "app"
});
con.connect(function(err) {
if (err) throw err;
con.query("SELECT * FROM info", function (err, result, fields) {
if (err) throw err;
console.log(result);
});
});
Code language: JavaScript (javascript)
Now run the below command to get the result-
node select.js
Code language: SQL (Structured Query Language) (sql)
Output-
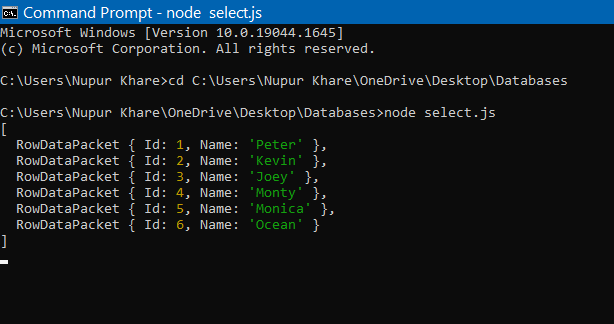
Here we can see that all the data records are retrieved.
Selecting specific columns using NodeJs
To just retrieve the Name field we will specify it. The javascript code for the following is-
var mysql = require('mysql');
var con = mysql.createConnection({
host: "localhost",
user: "root",
password: "******",
database: "app"
});
con.connect(function(err) {
if (err) throw err;
con.query("SELECT Name FROM info", function (err, result, fields) {
if (err) throw err;
console.log(result);
});
});
Code language: JavaScript (javascript)
Now run the following command to get results-
node select.js
Code language: SQL (Structured Query Language) (sql)
Output-
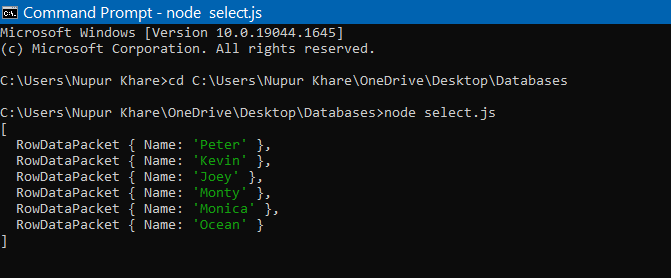
The Result Object
A result object is an array containing each row as an object. To get the Fourth record of the Name field from the table write the following-
var mysql = require('mysql');
var con = mysql.createConnection({
host: "localhost",
user: "root",
password: "******",
database: "app"
});
con.connect(function(err) {
if (err) throw err;
con.query("SELECT Name FROM info", function (err, result, fields) {
if (err) throw err;
console.log(result[3].Name);
});
});
Code language: JavaScript (javascript)
Output-
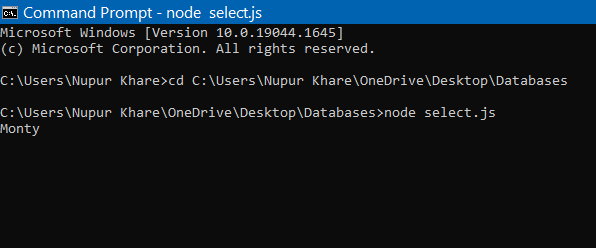
The Fields Object
The field object contains information about each field of the result set. To retrieve this the javascript code is as follows-
var mysql = require('mysql');
var con = mysql.createConnection({
host: "localhost",
user: "root",
password: "*****",
database: "app"
});
con.connect(function(err) {
if (err) throw err;
con.query("SELECT Id, Name FROM info", function (err, result, fields) {
if (err) throw err;
console.log(fields);
});
});
Code language: JavaScript (javascript)
Now run the command for getting results-
node select.js
Code language: SQL (Structured Query Language) (sql)
Output-
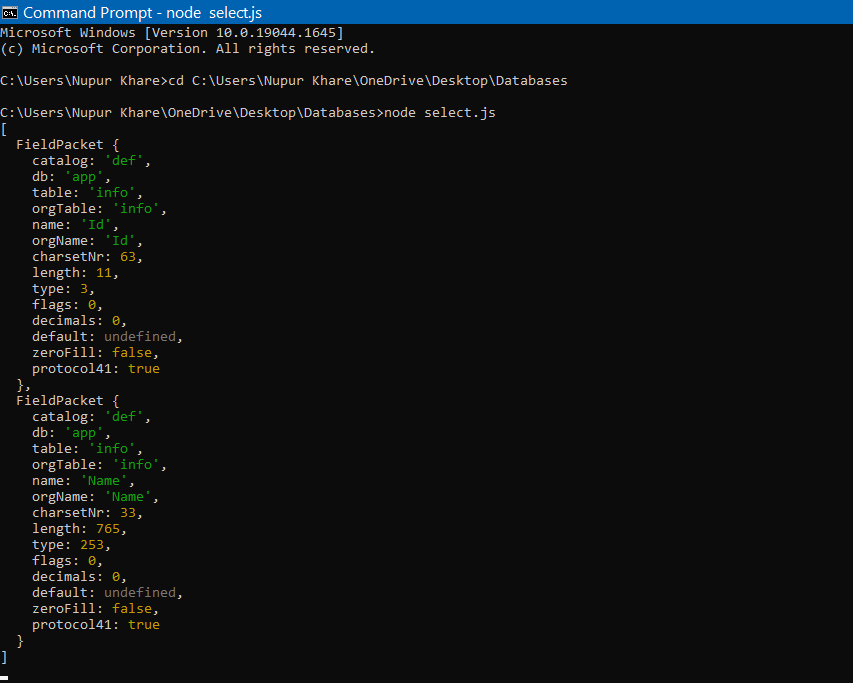
Here we can see that the detailed information has been retrieved.
Conclusion
In this tutorial, we learned how to use the SELECT statement to retrieve data from a MySQL database server using the Nodejs application.