We handle databases with the help of SQL queries. Complex problems require logical solutions. These logical solutions may be long sometimes. Problems based on data pipelines require very lengthy solutions. These types of queries are 1000 lines long in some cases. The long SQL queries contain different functions, clauses, and joins. Sometimes, queries also consist of some complex conditions and critical implementations. These queries should be handled properly. This article focuses on some tips and tricks to handle long SQL queries easily.
What Exactly Is a Long SQL Query?
All queries are not specified as a long query. Some characteristics of long queries distinguish them from normal/short queries. The long queries are always complex and contain critical logic that is hard to comprehend. The long queries consist of multiple joins, aggregate functions, some grouping, sorting, subqueries, and window functions. Now, you can imagine the complexity and level of logic required to implement these queries. Let’s try to understand this visually.
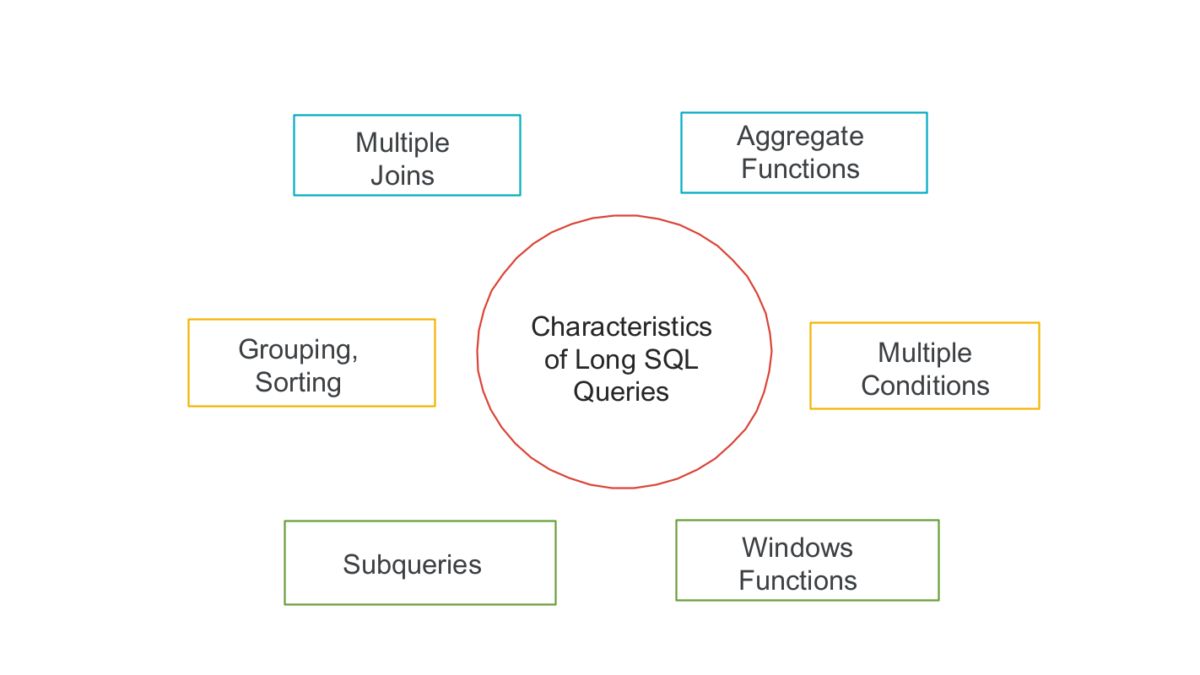
Tips for Organizing Long SQL Queries
Let’s discuss some techniques, tricks, and tips to manage long SQL queries simply. SQL language provides a wide range of functions, clauses, and different types of JOINs to minimize the complexity of the query. Let’s see this technique one by one.
1. Convert into Smaller and Logical Segments
The simple and easy way to manage a long query is to convert that query into smaller or logical components. The long queries can be divided into smaller segments using view. In the first example, we are using view/CTE to improve the readability of the overall query. The use of views also helps to maintain the query easily. For example, the logic is more visible in this case as compared to the original query (long queries).
CREATE VIEW Employee_Details AS
SELECT
employeeid_1 AS employee_id,
firstname_2 AS first_name,
lastname_3 AS last_name,
departmentid_4 AS department_id
FROM
Employees;
-- Create Department_Names view
CREATE VIEW Department_Names AS
SELECT
departmentid_1 AS department_id,
departmentname_2 AS department_name
FROM
Departments;
SELECT * FROM Employee_Details;
SELECT * FROM Department_Names;
Code language: SQL (Structured Query Language) (sql)
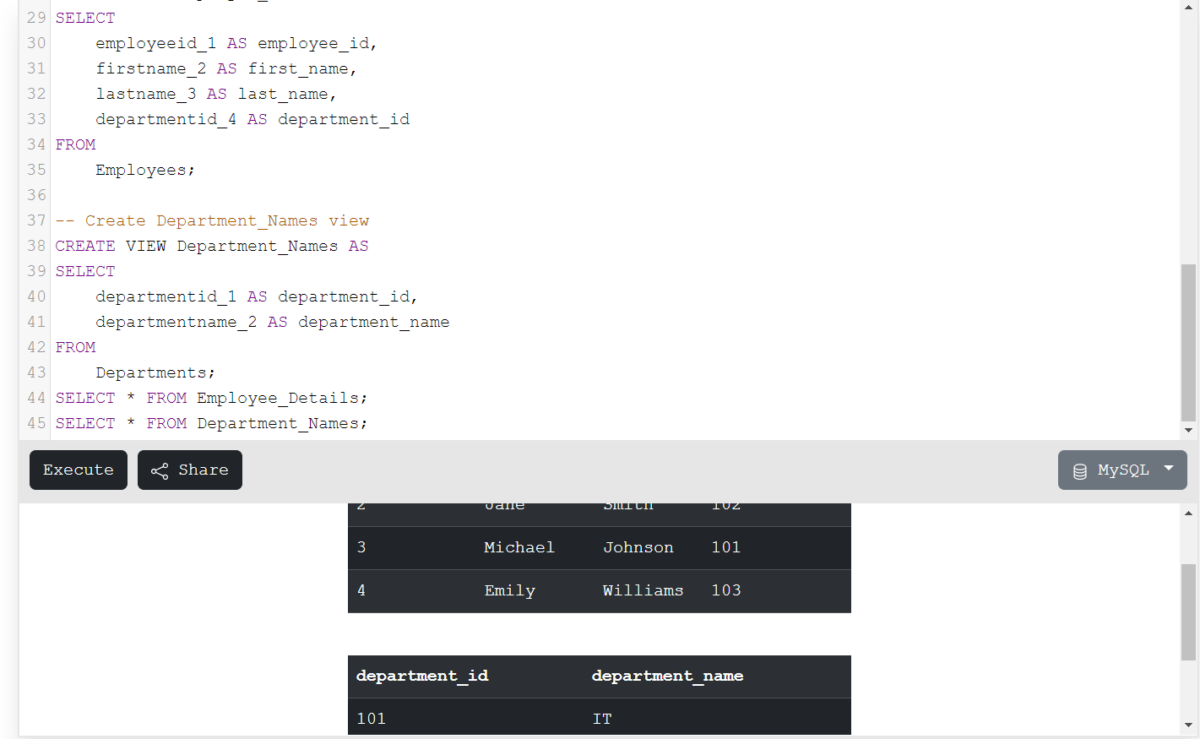
The above query is a sample query. You can apply this technique to make your long query more readable. The sample query is executed without any error.
2. Use of Meaningful Aliases
The main characteristics of the long query are multiple JOINs and Subqueries used in the query. The logic of these queries is always hard to understand and maintain. The second technique/trick we can use is to use meaningful aliases in the query for a clear understanding of the logic. The structure of queries becomes simple to understand.
SELECT
e.firstname_2 AS employees_firstname,
e.lastname_3 AS employees_lastname,
d.departmentname_2 AS department_info
FROM
Employees e
JOIN
Departments d ON e.departmentid_4 = d.departmentid_1;
Code language: SQL (Structured Query Language) (sql)
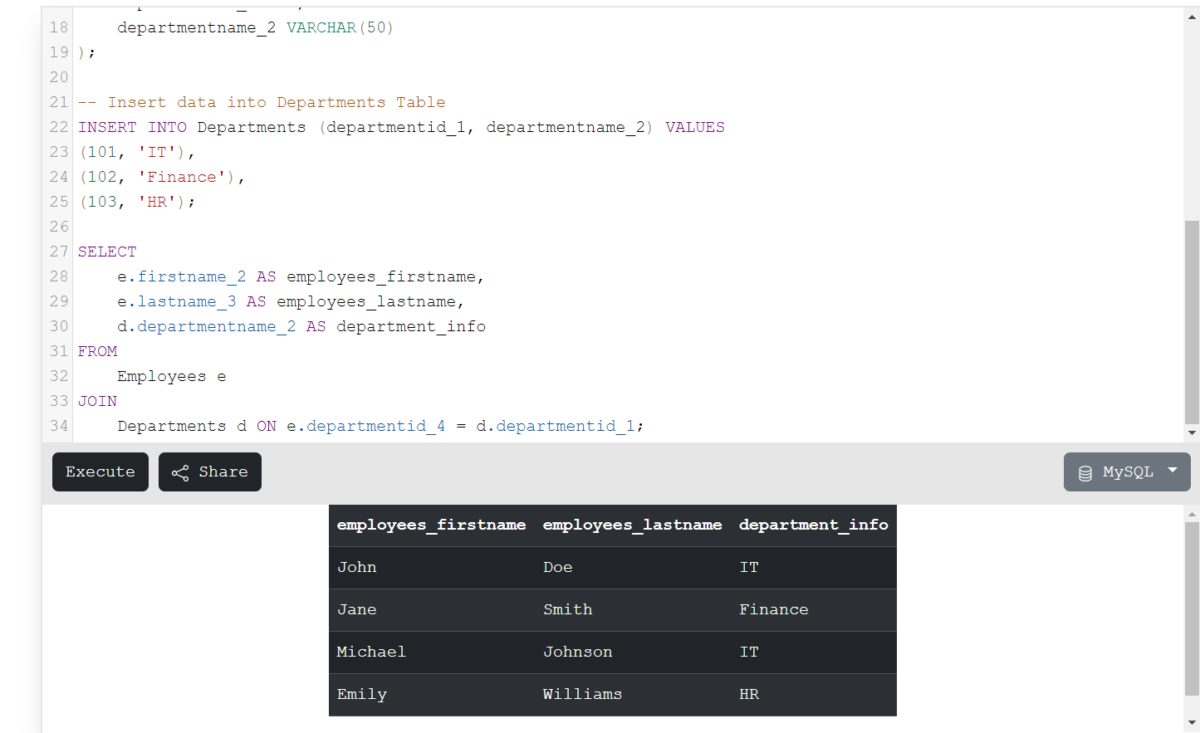
3. Use of Proper Indentation and Formatting
The indentation and accurate formatting of long SQL queries always help to maintain the code. The indentation of code improves readability. Accurate formatting of long SQL queries helps to understand the logic. This technique helps to manage the long SQL query easily. The accurate indentations and formatting also help to highlight every part of the query and the logic behind every step.
CREATE VIEW Employee_Details AS
SELECT
employeeid_1 AS employee_id,
firstname_2 AS first_name,
lastname_3 AS last_name,
departmentid_4 AS department_id
FROM
Employees;
SELECT * FROM Employee_Details;
Code language: SQL (Structured Query Language) (sql)
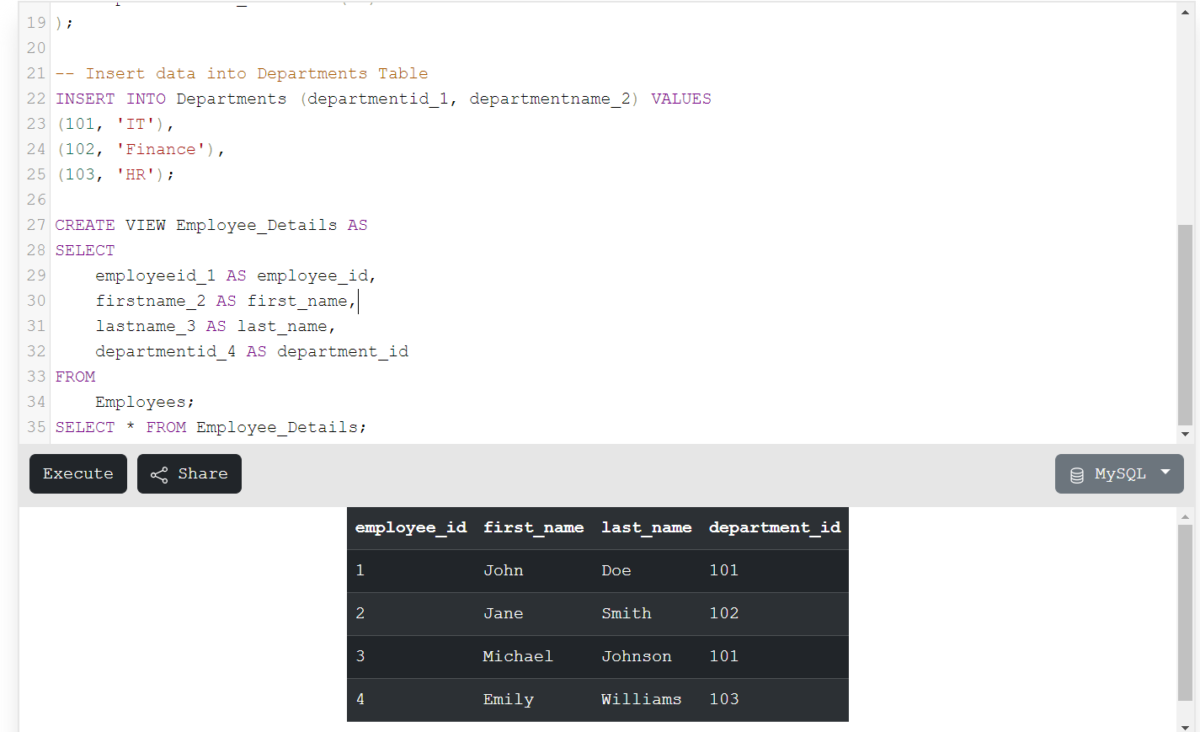
In the results, you can see the query is executed without any errors. This technique/trick is more useful when we deal with long queries with multiple joins and subqueries.
4. Use of Comments in Long SQL Queries
The comments in the long SQL queries help to document the logic behind every step. The long SQL queries always contain multiple JOINS, subqueries, and complex logic that need proper comments that help to explain the complex logic.
-- Create Department_Names view
CREATE VIEW Department_Names AS
SELECT
departmentid_1 AS department_id,
departmentname_2 AS department_name
FROM
Departments;
SELECT * FROM Department_Names;
Code language: SQL (Structured Query Language) (sql)
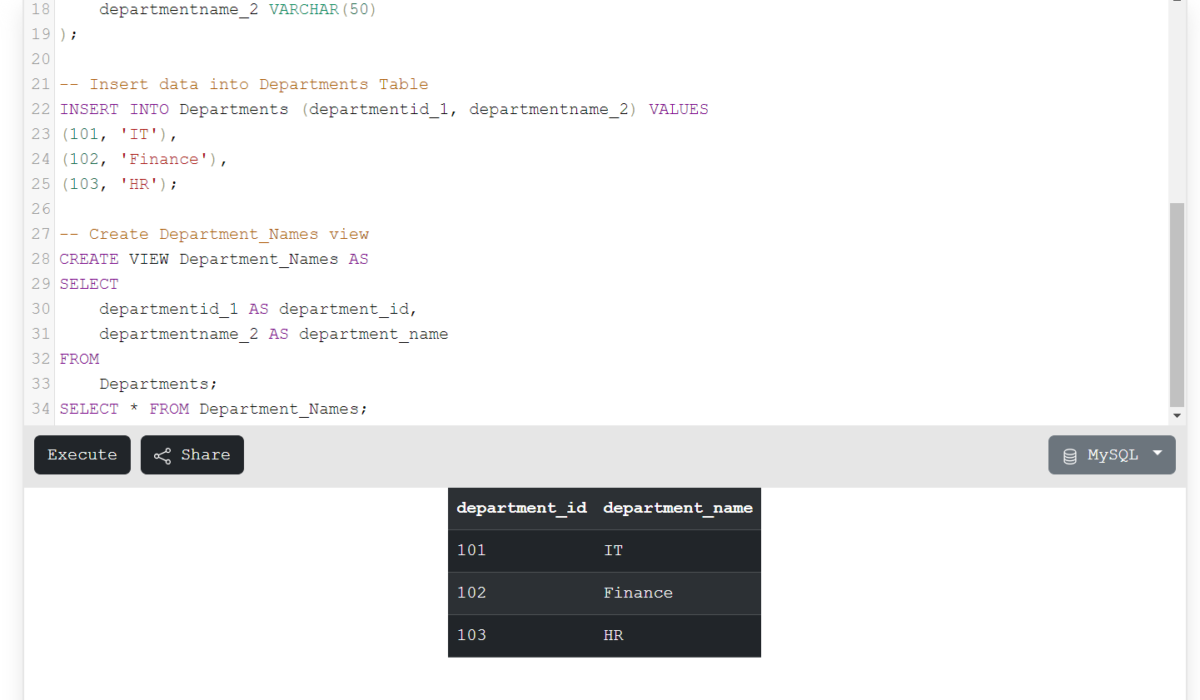
5. Testing and Validating Query for Accurate Results
The testing of long SQL query parts by parts is necessary to maintain the query and fix the errors. The logic of long SQL queries always depends on the accuracy of the query. Therefore, to maintain the long SQL queries we need to validate the queries from time to time. Validating the long SQL queries is a very simple and basic technique followed by every programmer to maintain the query.
-- Test join between employees and departments
SELECT
e.employeeid_1 AS employee_info,
e.firstname_2 AS firstname_info,
e.lastname_3 AS lastname_info,
d.departmentname_2 AS departmentname_info
FROM
Employees e
JOIN
Departments d ON e.departmentid_4 = d.departmentid_1;
Code language: SQL (Structured Query Language) (sql)
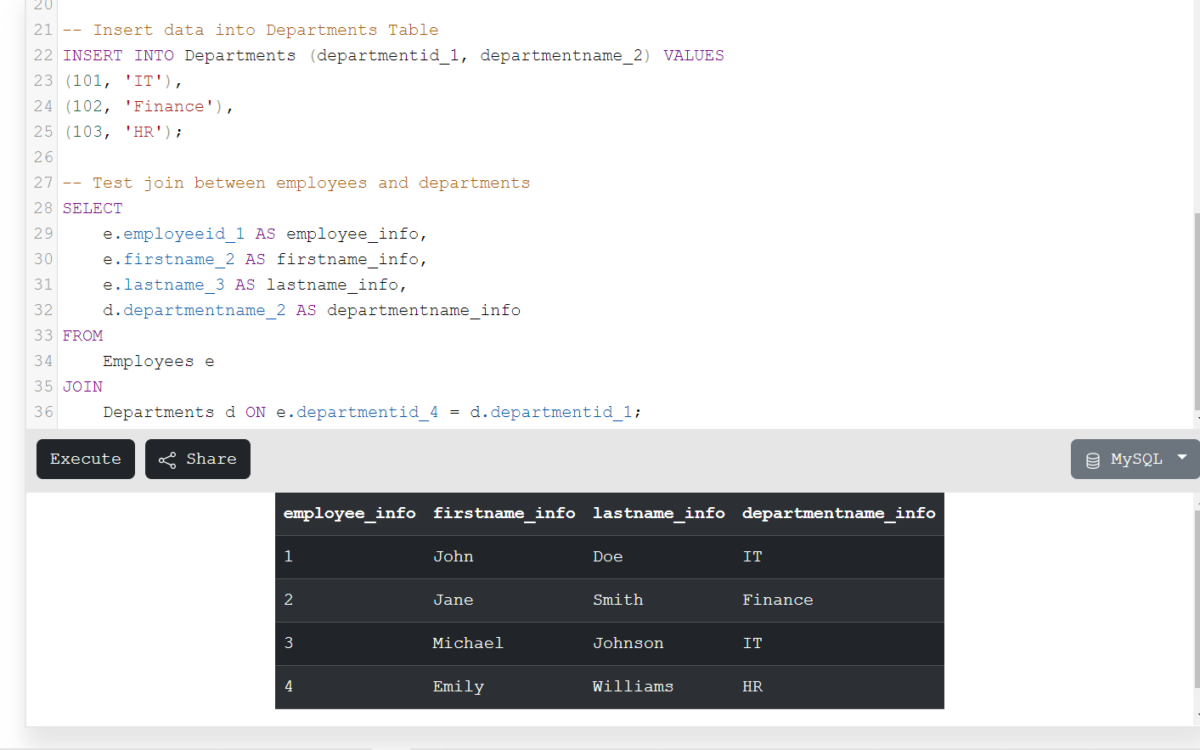
These are some techniques/tricks that help to maintain the long SQL queries effectively. This technique also helps to reduce the complexity and maintain the readability of the query.
Advantages of Organizing Long SQL Queries
Now that we know how to organize long SQL queries, let’s conclude this article by looking at some of its benefits.
1. Improved Performance
Increasing query performances is possible by optimizing long SQL queries. This implies proper indexing, tuning joins, and structuring queries to utilize the database’s facilities wisely. Well-planned queries run much faster, which lets the system respond faster to requests and helps to minimize the load on the database server.
2. Enhanced Readability and Maintainability
Effective handling of long SQL queries takes the form of grouping the statements and using the procedures as much as possible. As a result, this enhances the readability of developers and database administrators as it provides them an avenue to understand the query’s purpose and thus, it becomes easy to decipher its logic. Moreover, properly structured requests may be easily adapted and maintained, which therefore prevents malfunctions or errors during the updating and amendments processes.
3. Reduced Risk of Errors
Complex SQL queries can contain many misconceptions that lead to remaining tuned in the long queries and become evident in complex situations. With their management they can significantly decrease the likelihood of syntactic errors, unwarranted consistency gaps or issues on the performance side. This is provided through tough testing, code reviews, and compliance with standout principles of writing a query.
Summary
In this article, we have discussed some techniques and tricks to manage long SQL queries effectively. The long SQL queries always contain different functions, multiple joins, subqueries, and clauses. This article gives you a brief idea about the long SQL query and 5 effective techniques to manage long SQL queries. The advantages of managing long SQL queries are also explained in detail. Hope you will enjoy this article.
Reference
https://stackoverflow.com/questions/25186267/managing-very-large-sql-queries