In SQL, the GROUP BY clause is used to put together rows with the same values in some columns into simple groups. It’s often used with total functions like SUM(), COUNT(), AVG() and more to do tasks on every set of rows instead of all the results. The GROUP BY rule helps to join and make data easier in a computer database. It’s very effective! In this article, we will learn about 5 typical errors when using GROUP BY in SQL.
Common Errors While Writing GROUP BY Clause in SQL
When you use the GROUP BY clause in SQL, watch out for some usual mistakes. This is so your results are accurate and fit what you expect. Here are some common mistakes that people may make when writing a GROUP BY clause, along with examples. Let’s understand these errors using examples.
For this, we are using the same table for all the examples. This table contains some information related to sales, like date of sale, amount, date, etc. Let’s start with the table.
CREATE TABLE sales_table (
product_no INT,
date_of_sale DATE,
amount_spent DECIMAL(10, 2)
);
INSERT INTO sales_table VALUES
(1, '2024-01-01', 1000.00),
(1, '2024-01-01', 2000.00),
(2, '2024-01-01', 2500.00),
(2, '2024-01-02', 1200.00),
(3, '2024-01-02', 1800.00),
(3, '2024-01-02', 2000.00);
Code language: SQL (Structured Query Language) (sql)
We are using this table for every example.
1. Missing Function in GROUP BY Clause/Aggregate Function
In this example, two column names are mentioned in the SELECT clause but only one in the GROUP BY clause. We need to mention all the selected column names in the GROUP BY Clause or any aggregate function. Otherwise, this query will throw an error.
For example, date_of_sale is not included in the GROUP BY clause so it will throw an error. Always mention selected column names in GROUP BY or any aggregate function.
SELECT product_no, date_of_sale
FROM sales_table
GROUP BY product_no;
Code language: SQL (Structured Query Language) (sql)
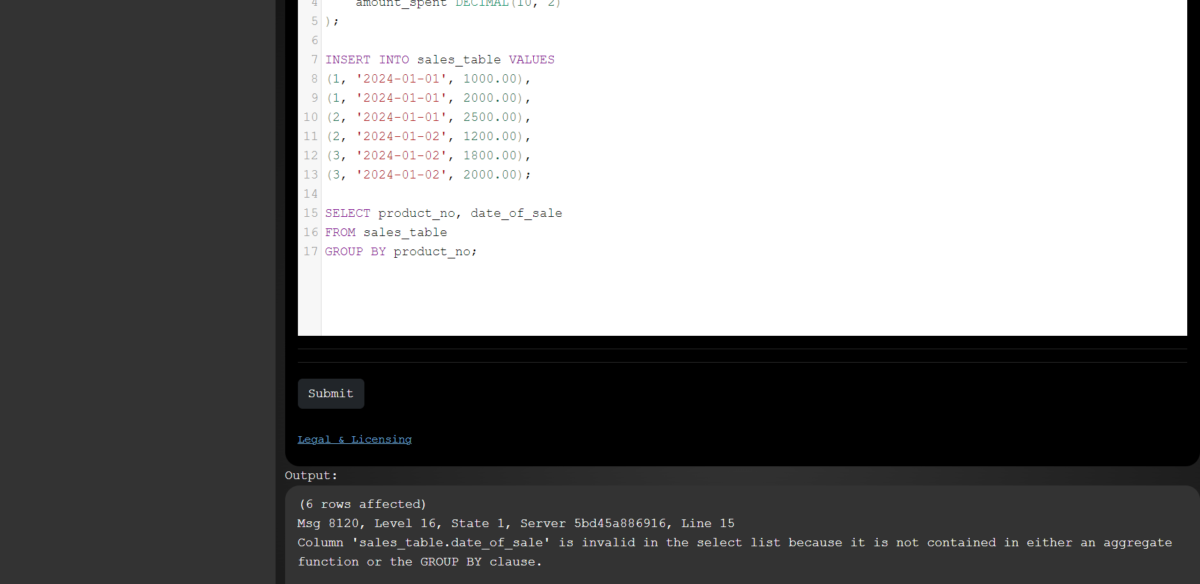
2. Using Aliases in GROUP BY Clause
In the GROUP BY clause, we can only use actual/real names for columns or expressions. The use of aliases will throw an error.
In this example, we have used max_amount in the SELECT list. This max_amount is not included in any GROUP BY or aggregate function.
SELECT product_id, MAX(amount) AS max_amount
FROM sales
GROUP BY max_amount;
Code language: SQL (Structured Query Language) (sql)
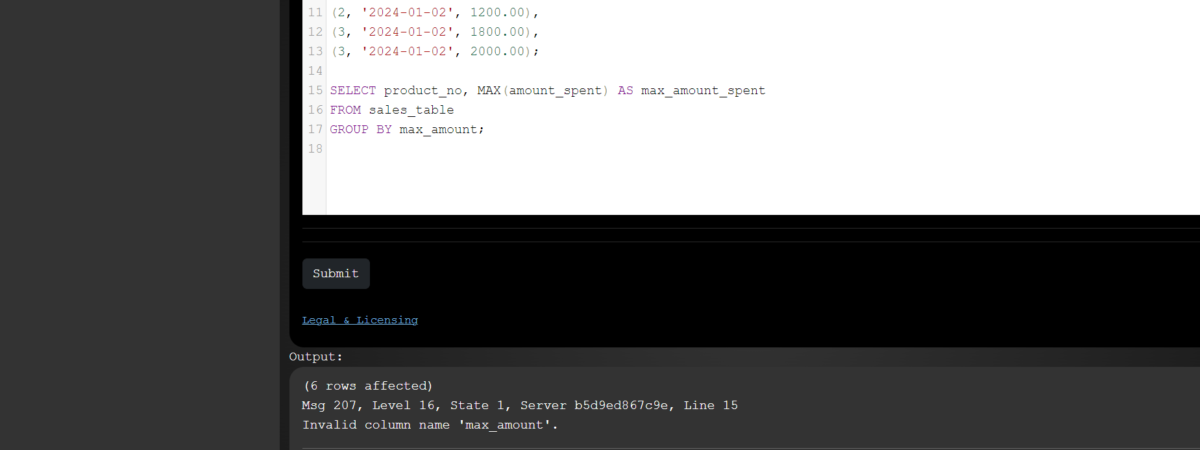
3. Aggregated and Non-Aggregated Columns in Query
Sometimes, the query contains both an aggregated function and a GROUP BY clause. One of them should contain the selected column that is mentioned in the query.
In the given example, we have used different columns in the SELECT statement, but the date_of_sale column is not mentioned in any aggregated function or GROUP BY clause.
SELECT product_no, MAX(amount_spent), date_of_sale
FROM sales_table
GROUP BY product_no;
Code language: SQL (Structured Query Language) (sql)
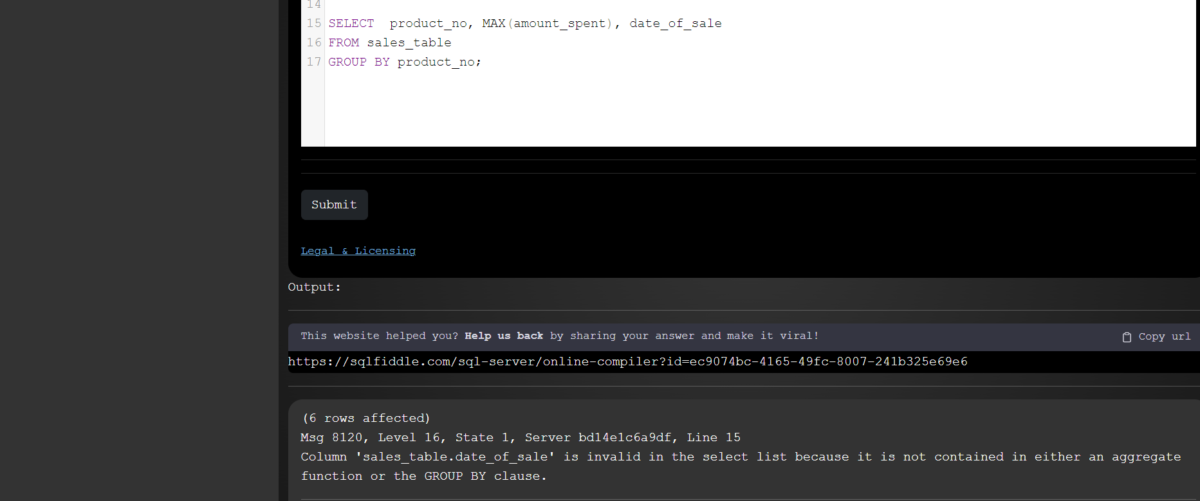
4. Incorrect Column References in Query
The rule of mentioning all the selected columns in any aggregate function or GROUP BY clause is also applicable to other clauses.
For example, if we are using the HAVING clause in our query and referring to the column name which is not mentioned in any GROUP BY clause or aggregated function then the query will throw an error.
SELECT product_no, MAX(amount_spent)AS max_amount
FROM sales_table
GROUP BY product_no
HAVING MAX(max_amount) > 1000;
Code language: SQL (Structured Query Language) (sql)
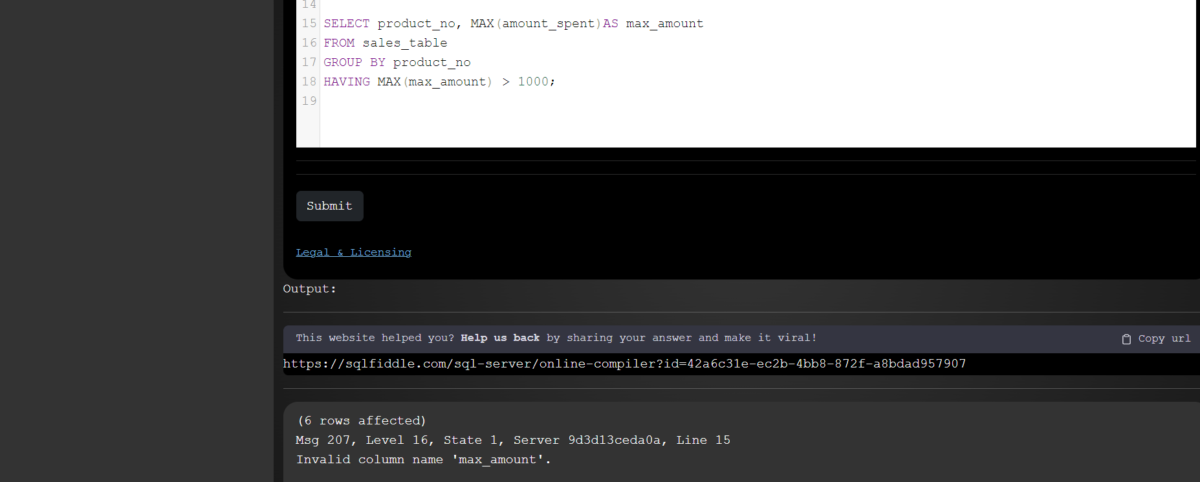
5. Applying GROUP BY on Invalid Columns
Sometimes, we use different column names that are not present in the table. This type of query will throw an error. The GROUP BY clause only use those columns that exist in the table.
In this example, let’s consider ABC_column as a non-existent column name. Now you can see the results.
SELECT ABC_column, MAX(amount_spent)
FROM sales_table
GROUP BY ABC_column;
Code language: SQL (Structured Query Language) (sql)
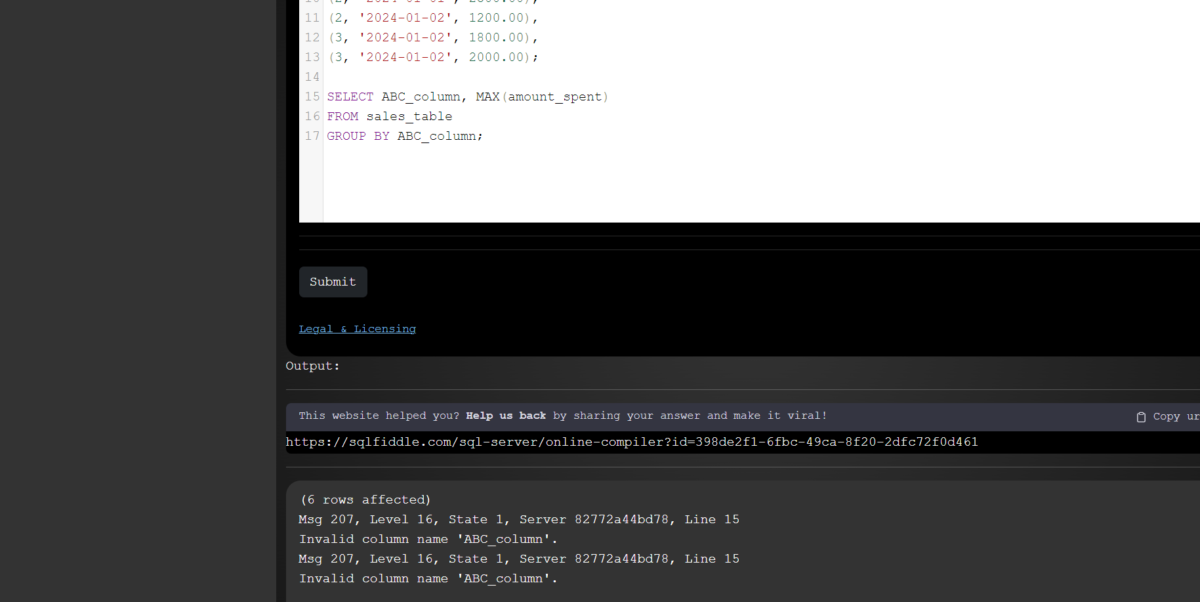
Benefits of Avoiding Mistakes in GROUP BY Clause
Not making errors when adding a GROUP BY part in SQL is very important. It makes sure the results you get from your query are correct and can be understood well.
- Accurate Aggregation: Using the GROUP BY clause properly make sure that information is added up correctly. Mistakes can cause wrong grouping outcomes, giving misleading details.
- Reliable Reporting: Making groups and adding them together correctly is needed for getting true reports. Not making errors in the GROUP BY section means your reports show real information about data.
- Consistent Data Analysis: Mistakes in the GROUP BY part can lead to a bad data study, which may cause incorrect conclusions. You make sure your thinking stays the same by not making errors.
- Preventing Data Leakage: Using the GROUP BY rightly stops information leaks by ensuring all non-added columns in the SELECT part are included in the GROUP BY part. It is very important to keep data safe and correct.
- Avoiding Performance Issues: Putting things in the wrong groups can lead to using up too many resources and speed issues. Using GROUP BY clauses well can make your queries faster, especially with lots of data.
Summary
In this article, we have looked at 5 typical errors that people make when using GROUP BY Clause in SQL. Don’t make errors with the GROUP BY part to get the right data groups, and good reports and keep all parts of your SQL query safe. It helps with doing tasks better, keeping code in good shape and following what business needs. The detailed benefits of not making errors when using the GROUP BY part in writing are explained clearly. We hope you enjoy this article.
Reference
https://stackoverflow.com/questions/16314836/group-by-clause-causing-error