In this tutorial, we will study how to delete data in MySQL using the Nodejs application.
Deleting MySQL records using Nodejs
To delete a record from the MySQL database server using the Nodejs application, the DELETE FROM statement will be used. For this, we will write a javascript code. Here table is info in the database app.
First, we will check the table info–
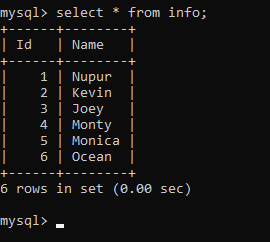
Execute this code by running the following command in the command prompt-
node delete.js
Code language: JavaScript (javascript)
Output-
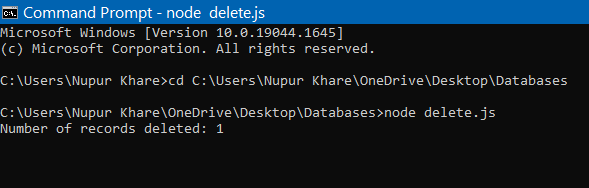
In the below code we replaced the record to be deleted with a placeholder ?.
var mysql = require('mysql');
var con = mysql.createConnection({
host: "localhost",
user: "root",
password: "*****",
database: "app"
});
con.connect(function(err) {
if (err) throw err;
var sql = "DELETE FROM info WHERE id = ?";
con.query(sql, function (err, result) {
if (err) throw err;
console.log("Number of records deleted: " + result.affectedRows);
});
});
Code language: JavaScript (javascript)
In the DELETE statement, we utilized the question mark (?) placeholder. We gave the data to the DELETE statement as the second argument when we called the query() function on the connection object to carry out the statement. When the query is executed, the input value will replace the placeholder, and ID will take the value 1.
Now we will again check whether the changes are made in the table info.
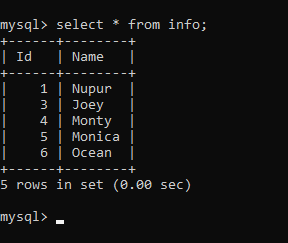
So here we can see that earlier there were 6 records and now there are 5 records. Hence, the changes have been made and the record is deleted.
An important point to remember here is that the WHERE clause is used with the DELETE FROM statement. This is so because the WHERE clause specifies which record or records are to be deleted. If the WHERE clause is omitted then all the records will be deleted!
Summary
In this tutorial, we studied how to delete records from a database using a Nodejs application.